PageLayout
Quickstart Examples
(All the examples given below assume that the static methods and constants of the class EasyCell
have been appropriately imported by using the import static pagelayout.EasyCell.*
statement.)
A Grid of Buttons
Baseline Alignment
An advanced example.
First Example
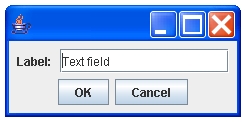
//Layout code Column topLevel=column(row(none,center,label,text), row(center,none,ok,cancel )); // Create the layout topLevel.createLayout(container);
//Detailed code // use import static pagelayout.EasyCell.* for import. // Create components JFrame frame=new JFrame(); Container container=frame.getContentPane(); JTextField text=new JTextField("Text field",15); JLabel label=new JLabel("Label: "); JButton ok= new JButton("OK"); JButton cancel= new JButton("Cancel"); // Top Row Column topLevel=column(row(none,center,label,text), row(center,none,ok,cancel )); // Create the layout topLevel.createLayout(container); frame.pack(); frame.show();
A Grid of Buttons
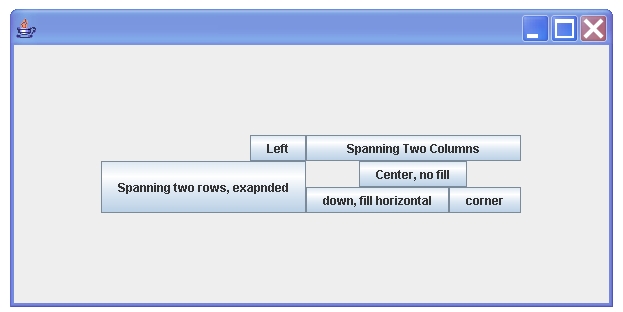
// Layout code // Create the layout. Cell topLevel =column(center,center, grid( row(right,none,leftButton), spantwocolumns, span(), eol(), spantworows, row(center,none,centernofill), span(), eol(), vspan(), downfillhoriz, corner)); // Make the required component sizes to be flexible. topLevel.setFixedWidth(false,downfillhoriz,spantwocolumns); topLevel.setFixedHeight(false,spantworows); // Set the component gaps to be zero topLevel.setComponentGaps(0,0); // Set the size of the grid to be fixed // after the components are laid out. topLevel.setFixedWidth(true,grid); topLevel.setFixedHeight(true,grid); // Create the layout. topLevel.createLayout(container)
JFrame frame=new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); Container container=frame.getContentPane(); // Create components JButton leftButton=new JButton("Left"); JButton spantwocolumns=new JButton("Spanning Two Columns"); JButton spantworows=new JButton("Spanning two rows, expanded"); JButton centernofill=new JButton("Center, no fill"); JButton downfillhoriz=new JButton("Down, fill horizontal"); JButton corner=new JButton("Corner"); // Create the layout. Cell topLevel= column(center,center, grid( row(right,none,leftButton), spantwocolumns, span(), eol(), spantworows, row(center,none,centernofill), span(), eol(), vspan(), downfillhoriz, corner)); // Make the required component sizes to be flexible. topLevel.setFixedWidth(false,downfillhoriz,spantwocolumns); topLevel.setFixedHeight(false,spantworows); // Set the component gaps to be zero topLevel.setComponentGaps(0,0); // Set the size of the grid to be fixed // after the components are laid out. topLevel.setFixedWidth(true,grid); topLevel.setFixedHeight(true,grid); // Create the layout. topLevel.createLayout(container) // pack and show frame.pack(); frame.show();
A Baseline Alignment Example
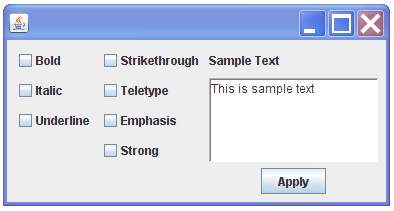
// Layout code CellGrid cellgrid= grid( bold, strikeThrough, sampleText, eol(), italic, teletype, column(center,none,textArea,apply), eol(), underline, emphasis, vspan(), eol(), skip(), strong, vspan()); // Baseline alignment of the components in the first row. cellgrid.alignBaseline(bold,strikeThrough,sampleText); // Create layout. cellgrid.createLayout(container);
// Detailed code JFrame frame=new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); Container container=frame.getContentPane(); // Create components JCheckBox bold=new JCheckBox("Bold"); JCheckBox italic=new JCheckBox("Italic"); JCheckBox underline=new JCheckBox("Underline"); JCheckBox strikeThrough=new JCheckBox("Strikethrough"); JCheckBox teletype=new JCheckBox("Teletype"); JCheckBox emphasis=new JCheckBox("Emphasis"); JCheckBox strong=new JCheckBox("Strong"); JLabel sampleText=new JLabel("Sample Text"); JButton apply=new JButton("Apply"); JTextArea textArea=new JTextArea("This is sample text"); textArea.setRows(5); textArea.setColumns(15); textArea.setBorder(BorderFactory.createLoweredBevelBorder()); CellGrid cellgrid= grid( bold, strikeThrough, sampleText, eol(), italic, teletype, column(center,none,textArea,apply), eol(), underline, emphasis, vspan(), eol(), skip(), strong, vspan()); // Baseline alignment of the components in the first row. cellgrid.alignBaseline(bold,strikeThrough,sampleText); // Create layout. cellgrid.createLayout(container); frame.pack(); frame.setSize(frame.getPreferredSize()); frame.show();
A slightly more complex Example

// Layout code CellGrid cellgrid=grid( dogName, dogNameEditor, span(), eol(), breed, combo, row(center,center,categories),eol(), photo, imagePanel, list, eol(), row(hgap(20),browse), vspan(), vspan(), eol(), row(hgap(20),delete), vspan(), vspan(), eol(), ownerInfoPanelCell(), span(), vspan(), eol(), row(right,center,enter),span(), span()); // Size constraints cellgrid.linkWidth(categories,2,list); cellgrid.linkWidth(browse,1,delete); // Fix the combo box height cellgrid.setFixedHeight(true,combo); // Make the list expandible in both directions. cellgrid.setFixedWidth(false,list); cellgrid.setFixedHeight(false,list); // Baseline alignments cellgrid.alignBaseline(dogName,dogNameEditor); cellgrid.alignBaseline(breed,combo,categories); // Create the layout cellgrid.createLayout(container);
Creation of OfficeInfoPanel for the grid shown above Cell gridcell=grid(name,nameEditor,eol(), phone, phoneEditor); // Baseline alignment gridcell.alignBaseline(name,nameEditor); gridcell.alignBaseline(phone,phoneEditor); // Create and return the panel cell return new PanelCell(panel,gridcell);
// Utility function for creating a JTextField with border public static JTextField createTextField(String text,int len) { JTextField ed=new JTextField(text,len); ed.setBorder(BorderFactory.createLoweredBevelBorder()); return ed; } public static PanelCell ownerInfoPanelCell() { // Create the cell for owner info editors // The panel JPanel panel=new JPanel(); Border border=BorderFactory.createEtchedBorder(); border=BorderFactory.createTitledBorder(border,"Owner Info"); panel.setBorder(border); // Create components JLabel name=new JLabel("Name"); JLabel phone=new JLabel("Phone"); JTextField nameEditor=createTextField("Jane Doe",10); JTextField phoneEditor=createTextField("555-3245",10); Cell gridcell=grid(name,nameEditor,eol(), phone, phoneEditor); // Baseline alignment gridcell.alignBaseline(name,nameEditor); gridcell.alignBaseline(phone,phoneEditor); // Create and return the panel cell return new PanelCell(panel,gridcell); } public static String [] breedCategories= { "Best of Breed", "Prettiest Female", "Handsomest Male", "Best Dressed", "Fluffiest Ears", "Most Colors", "Best Performer", "Loudest Bark", "Best Behaved", "Prettiest Eyes", "Most Hair", "Longest Tail", "Cutest Trick"}; public static String [] breeds= {"Collie", "Pitbull", "Poodle", "Scottie"}; public static void createGUI() { JFrame frame=new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); Container container=frame.getContentPane(); // Create Components JComboBox combo=new JComboBox(breeds); JLabel dogName=new JLabel("Dog's Name"); JTextField dogNameEditor=createTextField("Fifi",20); JLabel breed=new JLabel("Breed"); JLabel photo=new JLabel("Photo"); JLabel categories=new JLabel("Categories"); JButton browse=new JButton("Browse.."); JButton delete=new JButton("Delete"); JButton enter=new JButton("Enter"); JPanel imagePanel=new JPanel(); imagePanel.setBackground(new Color(200,200,255)); imagePanel.setBorder(BorderFactory.createLoweredBevelBorder()); JList list=new JList(breedCategories); list.setBorder(BorderFactory.createLoweredBevelBorder()); list.setBackground(Color.white); list.setVisibleRowCount(100); CellGrid cellgrid= grid(dogName, dogNameEditor,span(), eol(), breed, combo, row(center,center,categories), eol(), photo, imagePanel, list, eol(), row(hgap(20),browse), vspan(), vspan(), eol(), row(hgap(20),delete), vspan(), vspan(), eol(), ownerInfoPanelCell(), span(), vspan(), eol(), row(right,center,enter),span(),span()); // Size constraints cellgrid.linkWidth(categories,2,list); cellgrid.linkWidth(browse,1,delete); // Fix the combo box height cellgrid.setFixedHeight(true,combo); // Make the list expandible in both directions. cellgrid.setFixedWidth(false,list); cellgrid.setFixedHeight(false,list); // Baseline alignments cellgrid.alignBaseline(dogName,dogNameEditor); cellgrid.alignBaseline(breed,combo,categories); // Create the layout cellgrid.createLayout(container); frame.pack(); frame.setSize(frame.getPreferredSize()); frame.setVisible(true); }